Topological Optimization for Torus Genus Repair
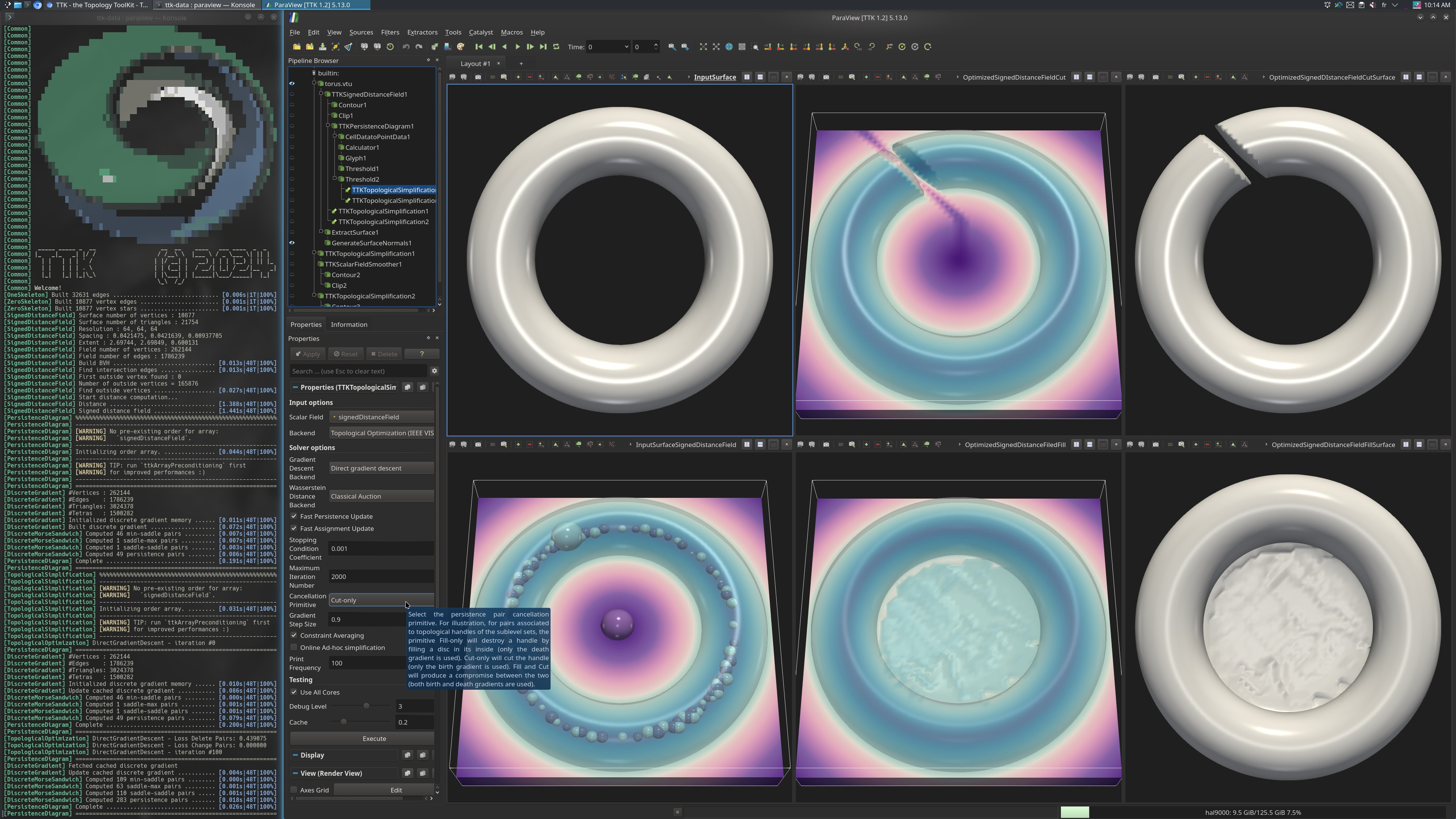
Pipeline description
This toy example simplifies the handle of a torus via the topological optimization of a SignedDistanceField to the input surface.
This processing pipeline first loads the surface.
Then, it computes a signed distance field to create a scalar field defined on a regular grid using the SignedDistanceField module.
The persistence diagram is computed using the PersistenceDiagram module and then it is thresholded to create a target diagram where only the persistence pair with infinite persistence is kept.
Finally, using the topological optimization backend of TopologicalSimplification, the pipeline optimizes the scalar field to remove the handle. This removal is exemplified with the cutting (bottom left) and filling (bottom right) strategies.
The python script computes the topological optimization and saves the output surfaces (for the cutting and filling strategies).
ParaView
To reproduce the above screenshot, go to your ttk-data directory and enter the following command:
paraview states/topologicalOptimization_torus.pvsm
Python code
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58 | #!/usr/bin/env python
from paraview.simple import *
# create a new 'XML Unstructured Grid Reader'
torusvtu = XMLUnstructuredGridReader(FileName=["torus.vtu"])
# create a new 'TTK SignedDistanceField'
tTKSignedDistanceField1 = TTKSignedDistanceField(Input=torusvtu)
tTKSignedDistanceField1.SamplingDimensions = [64, 64, 64]
# create a new 'TTK PersistenceDiagram'
tTKPersistenceDiagram1 = TTKPersistenceDiagram(Input=tTKSignedDistanceField1)
tTKPersistenceDiagram1.ScalarField = ["POINTS", "signedDistanceField"]
# create a new 'Threshold'
threshold2 = Threshold(Input=tTKPersistenceDiagram1)
threshold2.Scalars = ["CELLS", "IsFinite"]
# create a new 'TTK TopologicalSimplification'
tTKTopologicalSimplification1 = TTKTopologicalSimplification(
Domain=tTKSignedDistanceField1, Constraints=threshold2
)
tTKTopologicalSimplification1.ScalarField = ["POINTS", "signedDistanceField"]
tTKTopologicalSimplification1.Backend = "Topological Optimization (IEEE VIS 2024)"
tTKTopologicalSimplification1.StoppingConditionCoefficient = 0.001
tTKTopologicalSimplification1.MaximumIterationNumber = 2000
tTKTopologicalSimplification1.CancellationPrimitive = "Cut-only"
tTKTopologicalSimplification1.GradientStepSize = 0.9
# create a new 'TTK ScalarFieldSmoother'
tTKScalarFieldSmoother1 = TTKScalarFieldSmoother(Input=tTKTopologicalSimplification1)
tTKScalarFieldSmoother1.ScalarField = ["POINTS", "signedDistanceField"]
# create a new 'Contour'
contour1 = Contour(Input=tTKScalarFieldSmoother1)
contour1.ContourBy = ["POINTS", "signedDistanceField"]
contour1.Isosurfaces = [0.0]
# create a new 'TTK TopologicalSimplification'
tTKTopologicalSimplification2 = TTKTopologicalSimplification(
Domain=tTKSignedDistanceField1, Constraints=threshold2
)
tTKTopologicalSimplification2.ScalarField = ["POINTS", "signedDistanceField"]
tTKTopologicalSimplification2.Backend = "Topological Optimization (IEEE VIS 2024)"
tTKTopologicalSimplification2.StoppingConditionCoefficient = 0.001
tTKTopologicalSimplification2.MaximumIterationNumber = 2000
tTKTopologicalSimplification2.CancellationPrimitive = "Fill-only"
tTKTopologicalSimplification2.GradientStepSize = 0.9
tTKTopologicalSimplification2.ConstraintAveraging = 0
# create a new 'Contour'
contour2 = Contour(Input=tTKTopologicalSimplification2)
contour2.ContourBy = ["POINTS", "signedDistanceField"]
contour2.Isosurfaces = [0.0]
SaveData("topoOpt_torus_cut.vtp", contour1)
SaveData("topoOpt_torus_fill.vtp", contour2)
|
To run the above Python script, go to your ttk-data directory and enter the following command:
pvpython python/topologicalOptimization_torus.py
Outputs
topoOpt_torus_fill.vtp
: the repaired surface with the fill strategy.
topoOpt_torus_cut.vtp
: the repaired surface with the cut strategy.
C++/Python API
PersistenceDiagram
SignedDistanceField
TopologicalSimplification