Persistence Clustering 4
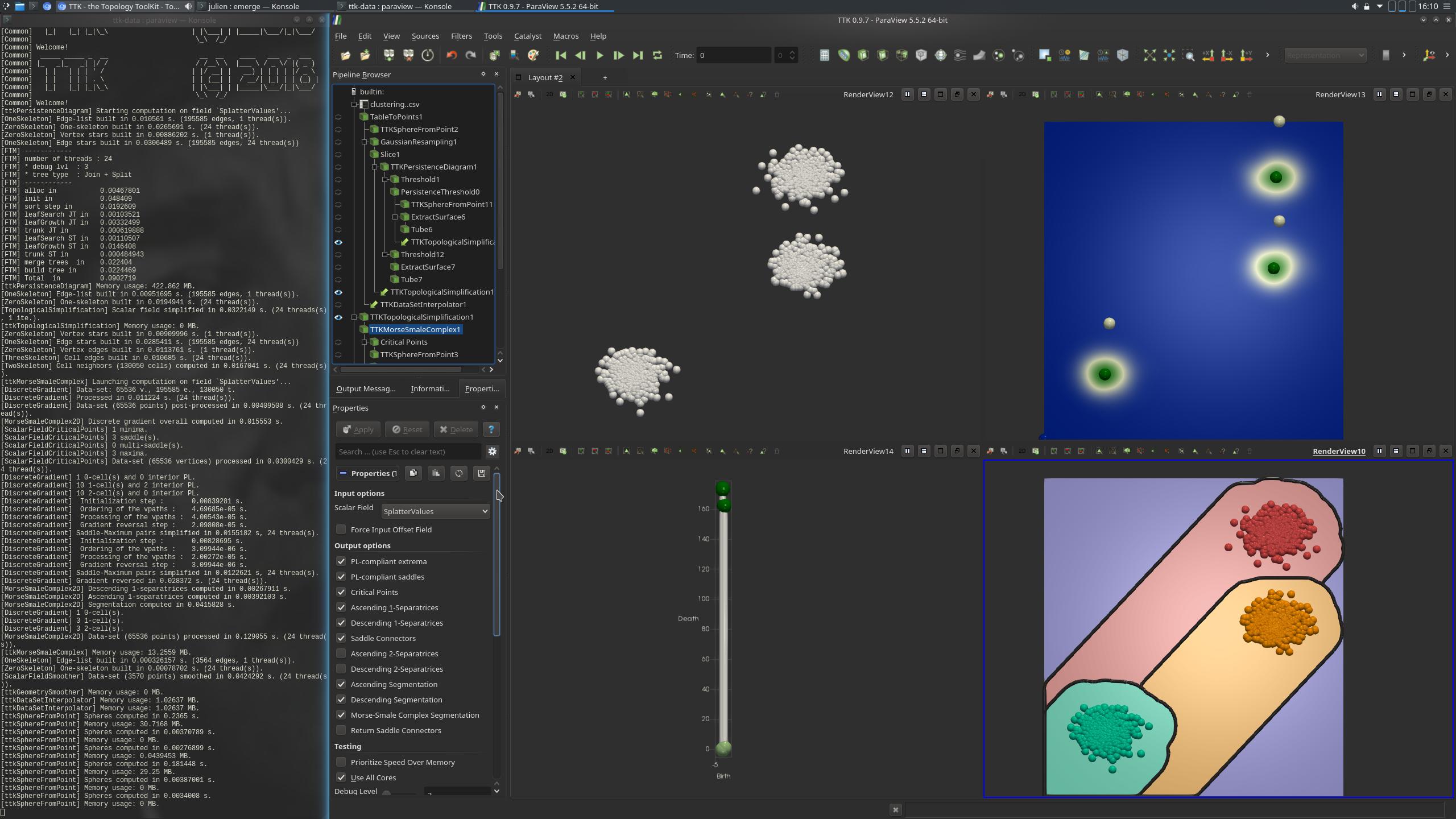
Pipeline description
This pipeline is the same as the previous ones and performs a clustering by persistence on a 2D data set taken from the scikit-learn examples. Please check out the Karhunen-Love Digits 64-Dimensions example for an application of this pipeline on a real-life data set..
First, this example loads a point cloud from disk (top left view in the above screenshot), then it computes a mesh on which a density field is obtained with a Gaussian Resampling on the points (top right view in the above screenshot). This density field will be considered as the input scalar data.
Next, a PersistenceDiagram is computed and thresholds are applied base on persistence to maintain only the features with a persistence above a certain value. The result is a simplified persistence diagram (bottom left view in the above screenshot).
The simplified persistence diagram is then used as a constraint for the TopologicalSimplification of the input scalar data, giving us a simplified data.
From there a MorseSmaleComplex is computed (bottom right view in the above screenshot). Finally, by using the identifier of the 2-dimension cell of the Morse Smale complex where one point lands, a cluster identifier, encoded in the AscendingManifold field in the ouput, is given to it.
ParaView
To reproduce the above screenshot, go to your ttk-data directory and enter the following command:
paraview states/persistenceClustering4.pvsm
Python code
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61 | from paraview.simple import *
# create a new 'CSV Reader'
clusteringcsv = CSVReader(FileName=["clustering4.csv"])
# create a new 'Table To Points'
tableToPoints1 = TableToPoints(Input=clusteringcsv)
tableToPoints1.XColumn = "X"
tableToPoints1.YColumn = "Y"
tableToPoints1.a2DPoints = 1
tableToPoints1.KeepAllDataArrays = 1
# create a new 'Gaussian Resampling'
gaussianResampling1 = GaussianResampling(Input=tableToPoints1)
gaussianResampling1.ResampleField = ["POINTS", "ignore arrays"]
gaussianResampling1.ResamplingGrid = [256, 256, 3]
gaussianResampling1.SplatAccumulationMode = "Sum"
# create a new 'Slice'
slice1 = Slice(Input=gaussianResampling1)
slice1.SliceType = "Plane"
# init the 'Plane' selected for 'SliceType'
slice1.SliceType.Normal = [0.0, 0.0, 1.0]
# create a new 'TTK PersistenceDiagram'
tTKPersistenceDiagram1 = TTKPersistenceDiagram(Input=slice1)
tTKPersistenceDiagram1.ScalarField = ["POINTS", "SplatterValues"]
# create a new 'Threshold'
threshold1 = Threshold(Input=tTKPersistenceDiagram1)
threshold1.Scalars = ["CELLS", "PairIdentifier"]
threshold1.ThresholdMethod = "Between"
threshold1.LowerThreshold = -0.1
threshold1.UpperThreshold = 999999999
# create a new 'Threshold'
persistenceThreshold0 = Threshold(Input=threshold1)
persistenceThreshold0.Scalars = ["CELLS", "Persistence"]
persistenceThreshold0.ThresholdMethod = "Between"
persistenceThreshold0.LowerThreshold = 10.0
persistenceThreshold0.UpperThreshold = 999999999
# create a new 'TTK TopologicalSimplification'
tTKTopologicalSimplification1 = TTKTopologicalSimplification(
Domain=slice1, Constraints=persistenceThreshold0
)
tTKTopologicalSimplification1.ScalarField = ["POINTS", "SplatterValues"]
# create a new 'TTK MorseSmaleComplex'
tTKMorseSmaleComplex1 = TTKMorseSmaleComplex(Input=tTKTopologicalSimplification1)
tTKMorseSmaleComplex1.ScalarField = ["POINTS", "SplatterValues"]
# create a new 'Resample With Dataset'
resampleWithDataset1 = ResampleWithDataset(
SourceDataArrays=OutputPort(tTKMorseSmaleComplex1, 3),
DestinationMesh=tableToPoints1,
)
# save the output(s)
SaveData("OutputClustering.csv", resampleWithDataset1)
|
To run the above Python script, go to your ttk-data directory and enter the following command:
pvpython python/persistenceClustering4.py
Outputs
data4Resampled.csv
: the output is the data resampled in CSV file format, the cluster identifier of a point is given in the AscendingManifold field.
C++/Python API
PersistenceDiagram
TopologicalSimplification
MorseSmaleComplex