Nested Tracking From Overlap
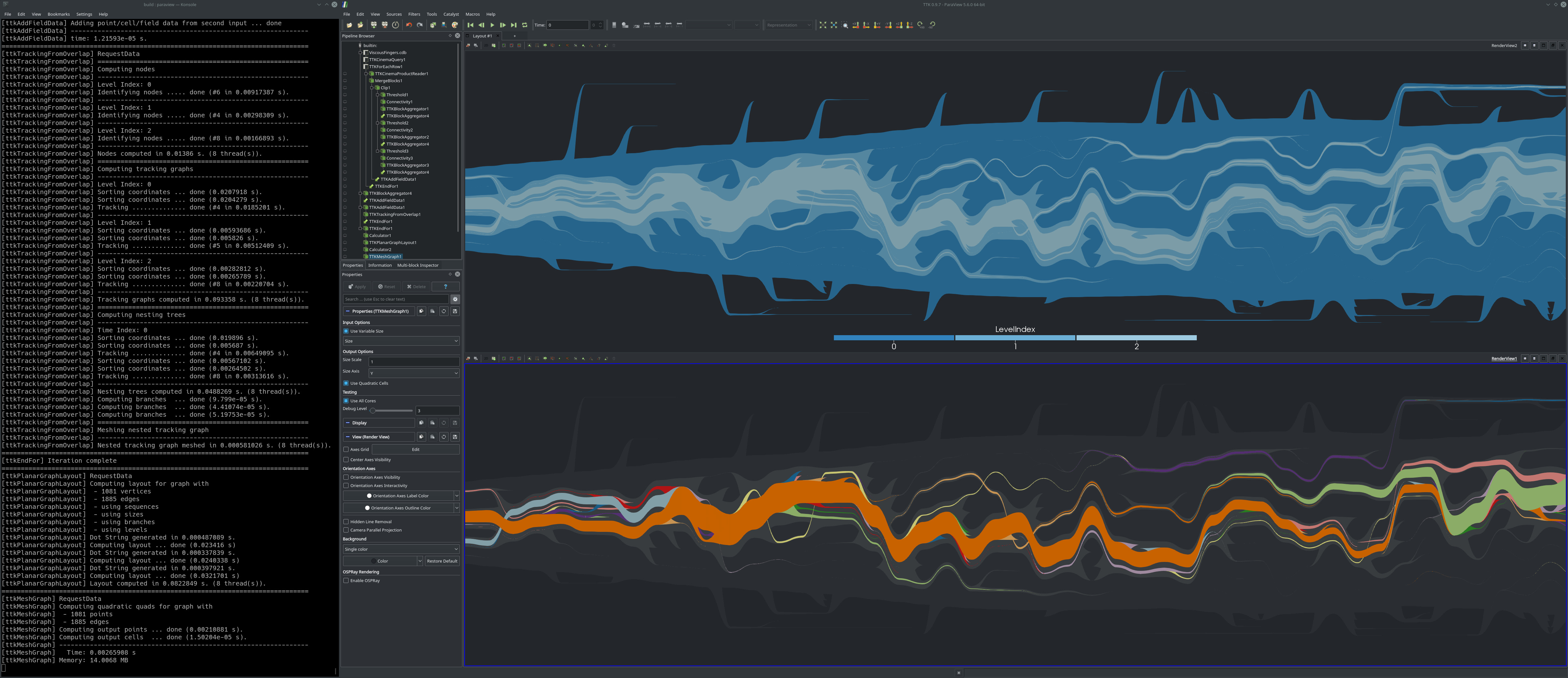
Pipeline description
This example first opens the viscous fingering cinema database via the ttkCinemaReader
, and then queries all entries of one specific simulation run ordered by time via the ttkCinemaQuery
filter.
Then the pipeline iterates over the resulting database entries via the ttkForEach
and ttkEndFor
filters, and loads the corresponding data product of each iteration with the ttkCinemaProductReader
.
Then the script computes three superlevel sets for the levels 20, 28, and 32 via three separate Threshold
filters.
Next the pipeline computs the connected components of these sets via the Connectivity
filter.
Then the components are packaged into a specific vtkMultiBlockDataSet
hierrachy via ttkBlockAggregator
filters, and this structure is then fed sequentially into the ttkTrackingFromOverlap
filter which computes the overlap of the components across time and levels.
After all iterations, the ttkTrackingFromOverlap
filter will produce the resulting nested tracking graph.
The ttkPlanarGraphLayout
filter then computes an optimized layout for the NTG, where the actual transformation of the graph coordinates is applied in the following Calculator
filter.
Finally the ttkMeshGraph
filter generates a vtkUnstructuredGrid
of the NTG with dynamic edge widths, which is then stored to disk.
ParaView
To reproduce the above screenshot, go to your ttk-data directory and enter the following command:
paraview states/nestedTrackingFromOverlap.py
Python code
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120 | #### import the simple module from the paraview
from paraview.simple import *
# create a new 'TTK CinemaReader'
ttkCinemaReader1 = TTKCinemaReader(DatabasePath="ViscousFingers.cdb")
# create a new 'TTK CinemaQuery'
ttkCinemaQuery1 = TTKCinemaQuery(InputTable=ttkCinemaReader1)
ttkCinemaQuery1.SQLStatement = """SELECT * FROM InputTable0
WHERE Sim='run01'
ORDER BY Time
LIMIT 100 OFFSET 20"""
# create a new 'TTK ForEach'
ttkForEach1 = TTKForEach(Input=ttkCinemaQuery1)
# create a new 'TTK CinemaProductReader'
ttkCinemaProductReader1 = TTKCinemaProductReader(Input=ttkForEach1)
# create a new 'Merge Blocks'
mergeBlocks1 = MergeBlocks(Input=ttkCinemaProductReader1)
# create a new 'Clip'
clip1 = Clip(Input=mergeBlocks1)
clip1.ClipType = "Plane"
clip1.HyperTreeGridClipper = "Plane"
clip1.Value = 38.38161849975586
# init the 'Plane' selected for 'ClipType'
clip1.ClipType.Origin = [31.5, 31.5, 50.0]
clip1.ClipType.Normal = [0.0, 0.0, 1.0]
# create a new 'Threshold'
threshold1 = Threshold(Input=clip1)
threshold1.Scalars = ["POINTS", "ImageFile"]
threshold1.ThresholdMethod = "Above Upper Threshold"
threshold1.UpperThreshold = 20
# create a new 'Threshold'
threshold2 = Threshold(Input=clip1)
threshold2.Scalars = ["POINTS", "ImageFile"]
threshold2.ThresholdMethod = "Above Upper Threshold"
threshold2.UpperThreshold = 28
# create a new 'Threshold'
threshold3 = Threshold(Input=clip1)
threshold3.Scalars = ["POINTS", "ImageFile"]
threshold3.ThresholdMethod = "Above Upper Threshold"
threshold3.UpperThreshold = 32
# create a new 'Connectivity'
connectivity1 = Connectivity(Input=threshold1)
# create a new 'Connectivity'
connectivity2 = Connectivity(Input=threshold2)
# create a new 'Connectivity'
connectivity3 = Connectivity(Input=threshold3)
# create a new 'TTK BlockAggregator'
ttkBlockAggregator5 = TTKBlockAggregator(Input=connectivity1)
ttkBlockAggregator5.ForceReset = 1
# create a new 'TTK BlockAggregator'
ttkBlockAggregator6 = TTKBlockAggregator(Input=connectivity2)
ttkBlockAggregator6.ForceReset = 1
# create a new 'TTK BlockAggregator'
ttkBlockAggregator7 = TTKBlockAggregator(Input=connectivity3)
ttkBlockAggregator7.ForceReset = 1
# create a new 'TTK BlockAggregator'
ttkBlockAggregator1 = TTKBlockAggregator(
Input=[ttkBlockAggregator5, ttkBlockAggregator6, ttkBlockAggregator7]
)
ttkBlockAggregator1.ForceReset = 1
ttkBlockAggregator1.FlattenInput = 0
# create a new 'TTK ArrayEditor'
ttkArrayEditor1 = TTKArrayEditor(
Target=ttkBlockAggregator1, Source=ttkCinemaProductReader1
)
ttkArrayEditor1.EditorMode = "Add Arrays from Source"
ttkArrayEditor1.TargetAttributeType = "Field Data"
ttkArrayEditor1.SourceFieldDataArrays = ["_ttk_IterationInfo"]
# create a new 'TTK TrackingFromOverlap'
ttkTrackingFromOverlap1 = TTKTrackingFromOverlap(Input=ttkArrayEditor1)
ttkTrackingFromOverlap1.Labels = "RegionId"
# create a new 'TTK EndFor'
ttkEndFor1 = TTKEndFor(Data=ttkTrackingFromOverlap1, For=ttkForEach1)
# create a new 'Calculator'
calculator1 = Calculator(Input=ttkEndFor1)
calculator1.ResultArrayName = "Size"
calculator1.Function = "Size/6000"
calculator1.ResultArrayType = "Float"
# create a new 'TTK PlanarGraphLayout'
ttkPlanarGraphLayout1 = TTKPlanarGraphLayout(Input=calculator1)
ttkPlanarGraphLayout1.UseSequences = 1
ttkPlanarGraphLayout1.SequenceArray = ["POINTS", "SequenceIndex"]
ttkPlanarGraphLayout1.UseSizes = 1
ttkPlanarGraphLayout1.SizeArray = ["POINTS", "Size"]
ttkPlanarGraphLayout1.UseBranches = 1
ttkPlanarGraphLayout1.BranchArray = ["POINTS", "BranchId"]
ttkPlanarGraphLayout1.UseLevels = 1
ttkPlanarGraphLayout1.LevelArray = ["POINTS", "LevelIndex"]
# create a new 'Calculator'
calculator2 = Calculator(Input=ttkPlanarGraphLayout1)
calculator2.CoordinateResults = 1
calculator2.Function = "iHat*SequenceIndex+jHat*Layout_Y+kHat*LevelIndex"
# create a new 'TTK MeshGraph'
ttkMeshGraph1 = TTKMeshGraph(Input=calculator2)
ttkMeshGraph1.SizeArray = ["POINTS", "Size"]
SaveData("NestedTrackingGraph.vtu", ttkMeshGraph1)
|
To run the above Python script, go to your ttk-data directory and enter the following command:
pvpython python/nestedTrackingFromOverlap.py
Outputs
NTG.vtu
: the meshed nested tracking graph of superlevel set components for the levels 20, 28, and 32.
C++/Python API
ArrayEditor
BlockAggregator
CinemaProductReader
CinemaQuery
CinemaReader
EndFor
ForEach
MeshGraph
PlanarGraphLayout
TrackingFromOverlap